Servlet实现文件上传
由于步骤相对比较固定,所以本文只记录核心步骤,其他步骤一般可以想出来,想不出来去翻这一篇博客:servlet-文件上传-狂神笔记 - 你我不在年少 - 博客园 (cnblogs.com)
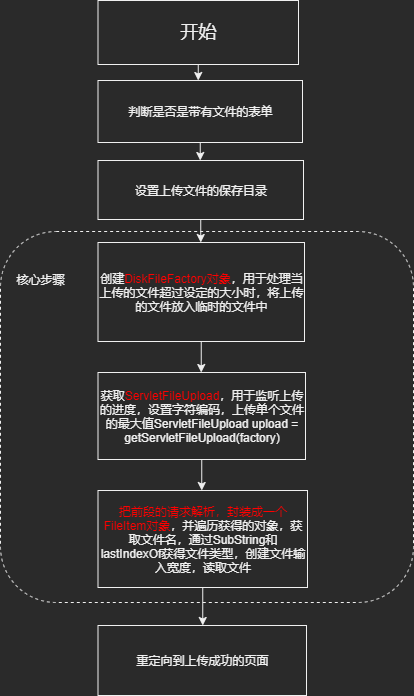
步骤一:导入包
①commons-io依赖
②commons-fileupload依赖
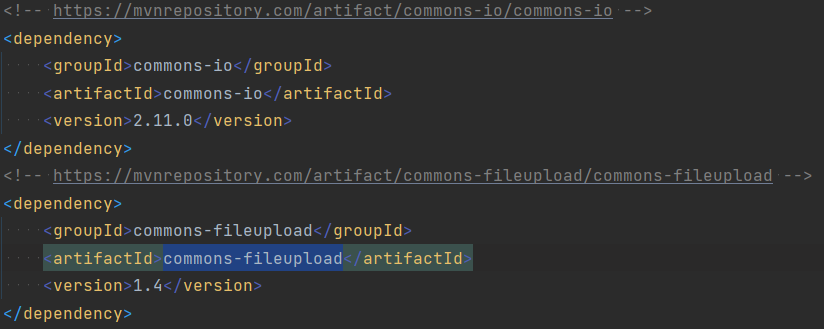
步骤二:获取DiskFileItemFactory对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
|
public static DiskFileItemFactory getDiskFileItemFactory(File tempfile) { DiskFileItemFactory factory = new DiskFileItemFactory();
factory.setSizeThreshold(1024 * 1024 * 2); factory.setRepository(tempfile);
return factory; }
|
步骤三:获取ServletFileUpload对象
构造方法中可以传入步骤二中获取到的DiskFileItemFactory对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
|
public static ServletFileUpload getServletFileUpload(DiskFileItemFactory factory) { ServletFileUpload upload = new ServletFileUpload(factory);
upload.setHeaderEncoding("utf-8"); upload.setFileSizeMax(1024 * 1024 * 100); upload.setSizeMax(1024 * 1024 * 100);
upload.setProgressListener(new ProgressListener() { @Override public void update(long pBytesRead, long pContentLength, int pItems) { System.out.println("总大小:" + pContentLength + " 已上传" + pBytesRead); } });
return upload; }
|
步骤三:解析请求,获取文件,返回结果
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| public String parseUploadRequest(HttpServletRequest request, ServletFileUpload upload, String uploadPath) throws FileUploadException, IOException { List<FileItem> fileItems = upload.parseRequest(request); String msg = "";
for (FileItem fileItem : fileItems) { if (fileItem.isFormField()) { continue; } else { if (fileItem.getName().trim().equals("") || fileItem.getName() == null) { continue; } else { String uploadFileName = fileItem.getName(); System.out.println("uploadFileName" + uploadFileName);
String fileName = uploadFileName.substring(uploadFileName.lastIndexOf("/") + 1); System.out.println("fileName:" + fileName); String fileExtName = uploadFileName.substring(uploadFileName.lastIndexOf(".") + 1); System.out.println("fileExtName:" + fileExtName);
InputStream inputStream = fileItem.getInputStream(); String uuidStirng = UUID.randomUUID().toString(); FileOutputStream fos = new FileOutputStream(uploadPath + "/" + uuidStirng + "." + fileExtName);
byte[] buffer = new byte[1024 * 1024];
while (inputStream.read(buffer) > 0) { fos.write(buffer); }
fos.flush(); fos.close();
inputStream.close(); msg = "文件上传成功"; } } fileItem.delete(); } return msg; }
|
小结
步骤相对固定。但其中还会有些许其他小技巧,如:获取文件名、借助UUID编号保持文件名唯一性。